

1 /*
2 * TextureManager.h
3 *
4 * Created on: 2014年12月13日
5 * Author: wgzll
6 */
7
8 #ifndef SRC_TEXTUREMANAGER_H_
9 #define SRC_TEXTUREMANAGER_H_
10
11 #include <iostream>
12 #include <string>
13 #include <map>
14 #include <sdl2/sdl.h>
15
16 using namespace std;
17
18 class TextureManager
19 {
20 public:
21 static TextureManager* Instance()
22 {
23 if(s_pInstance == 0)
24 {
25 s_pInstance = new TextureManager();
26 return s_pInstance;
27 }
28
29 return s_pInstance;
30 }
31
32 bool load(string fileName,string id,SDL_Renderer* pRenderer);
33 void draw(string id,int x,int y,int width,int height,SDL_Renderer* pRenderer,SDL_RendererFlip flip=SDL_FLIP_NONE);
34 void drawFrame(string id,int x,int y,int width,int height,int currentRow,int currentFrame,SDL_Renderer* pRenderer,double angle,int alpha,SDL_RendererFlip flip=SDL_FLIP_NONE);
35
36 map<string,SDL_Texture*> getTextureMap(){return m_textureMap;}
37
38 public:
39 TextureManager(){}
40 ~TextureManager(){}
41 private:
42 map<string,SDL_Texture*> m_textureMap;
43 static TextureManager* s_pInstance;
44
45 };
46
47 typedef TextureManager TheTextureManager;
48
49
50 #endif /* SRC_TEXTUREMANAGER_H_ */
51


1 /*
2 * TextureManager.cpp
3 *
4 * Created on: 2014年12月13日
5 * Author: wgzll
6 */
7
8 #include "TextureManager.h"
9 #include <sdl2/sdl.h>
10 #include <sdl2/sdl_image.h>
11
12 TextureManager* TextureManager::s_pInstance = 0;
13
14 bool TextureManager::load(string fileName,string id,SDL_Renderer* pRenderer)
15 {
16 SDL_Surface* pTempSurface = IMG_Load(fileName.c_str());
17
18
19 if(pTempSurface == 0)
20 {
21 cout<<IMG_GetError();
22 return false;
23 }
24
25 SDL_Texture* pTexture = SDL_CreateTextureFromSurface(pRenderer,pTempSurface);
26
27 SDL_FreeSurface(pTempSurface);
28
29 if(pTexture != 0)
30 {
31 m_textureMap[id] = pTexture;
32 return true;
33 }
34 return false;
35 }
36
37 void TextureManager::draw(string id, int x, int y, int width, int height, SDL_Renderer* pRenderer, SDL_RendererFlip flip)
38 {
39 SDL_Rect srcRect;
40 SDL_Rect destRect;
41
42 srcRect.x = 0;
43 srcRect.y = 0;
44 srcRect.w = destRect.w = width;
45 srcRect.h = destRect.h = height;
46 destRect.x = x;
47 destRect.y = y;
48
49 SDL_RenderCopyEx(pRenderer, m_textureMap[id], &srcRect, &destRect, 0, 0, flip);
50 }
51
52 void TextureManager::drawFrame(string id, int x, int y, int width, int height, int currentRow, int currentFrame, SDL_Renderer *pRenderer, double angle, int alpha, SDL_RendererFlip flip)
53 {
54 SDL_Rect srcRect;
55 SDL_Rect destRect;
56 srcRect.x = width * currentFrame;
57 srcRect.y = height * currentRow;
58 srcRect.w = destRect.w = width;
59 srcRect.h = destRect.h = height;
60 destRect.x = x;
61 destRect.y = y;
62
63 SDL_SetTextureAlphaMod(m_textureMap[id], alpha);
64 SDL_RenderCopyEx(pRenderer, m_textureMap[id], &srcRect, &destRect, angle, 0, flip);
65 }
66
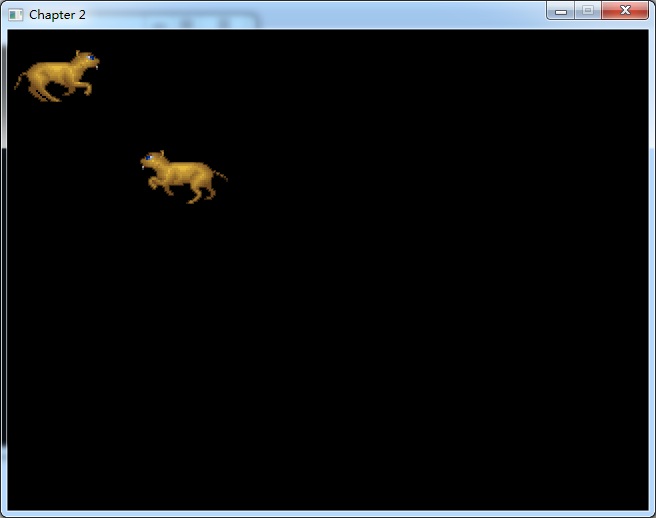